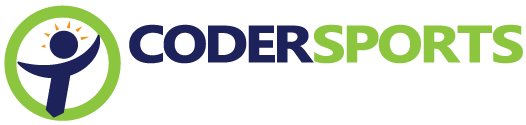
In the make tasks, students use the skills learned in the earlier stages of PRIMM to create their own program based on a description of what it should do.
Make sure that the students add comments to explain what the code does.
Advanced If…Else Statements
Validation is the process of checking whether data is sensible and allowable. It’s a really important part of lots of programs that involve input (for example checking your password).
We can either check if a number is inside a certain range, or outside a certain range.
To check if a number is within a range, we use:
Greater than or equal to the lowest value AND less than or equal to the highest value.
Both conditions have to be True to ensure that the number is within the range.
num_1 = input("Enter a number between 1 and 10")
if num_1 >= 1 and num_1 <= 10:
print("Number in range")
else:
print("Number not in range")
To check if a number is outside a range, we use:
Less than the lowest value OR greater than the highest value.
Only one condition has to be True to ensure that the number is outside the range. It would be impossible for both conditions to be True
num_1 = input("Enter a number between 1 and 10")
if num_1 < 1 or num_1 > 10:
print("Number not in range")
else:
print("Number in range")
Help! My Code Does Not Work!
Make sure to look out for the following things:
- There is a full condition (data, operator, data) on both sides of the Boolean operator (
and
,or
).
- The correct variable is being checked
-
and
is used for inside a range
-
or
is used for outside a range